2018-04-07 13:49:12 +00:00
<!-- page_number: true -->

----
# 
# Une formation par Klafyvel et Nanoy2
----
# Qu'est-ce que Django peut faire ?
----
# Qu'est-ce que Django peut faire ?
- coope.rez
- Re2o
- Blogs
- ...
2018-04-10 11:23:12 +00:00
---

---

---

---

2018-04-07 13:49:12 +00:00
----
# Qu'est-ce que Django ne peut pas faire ?
---
# Qu'est-ce que Django ne peut pas faire ?
- Rien
---
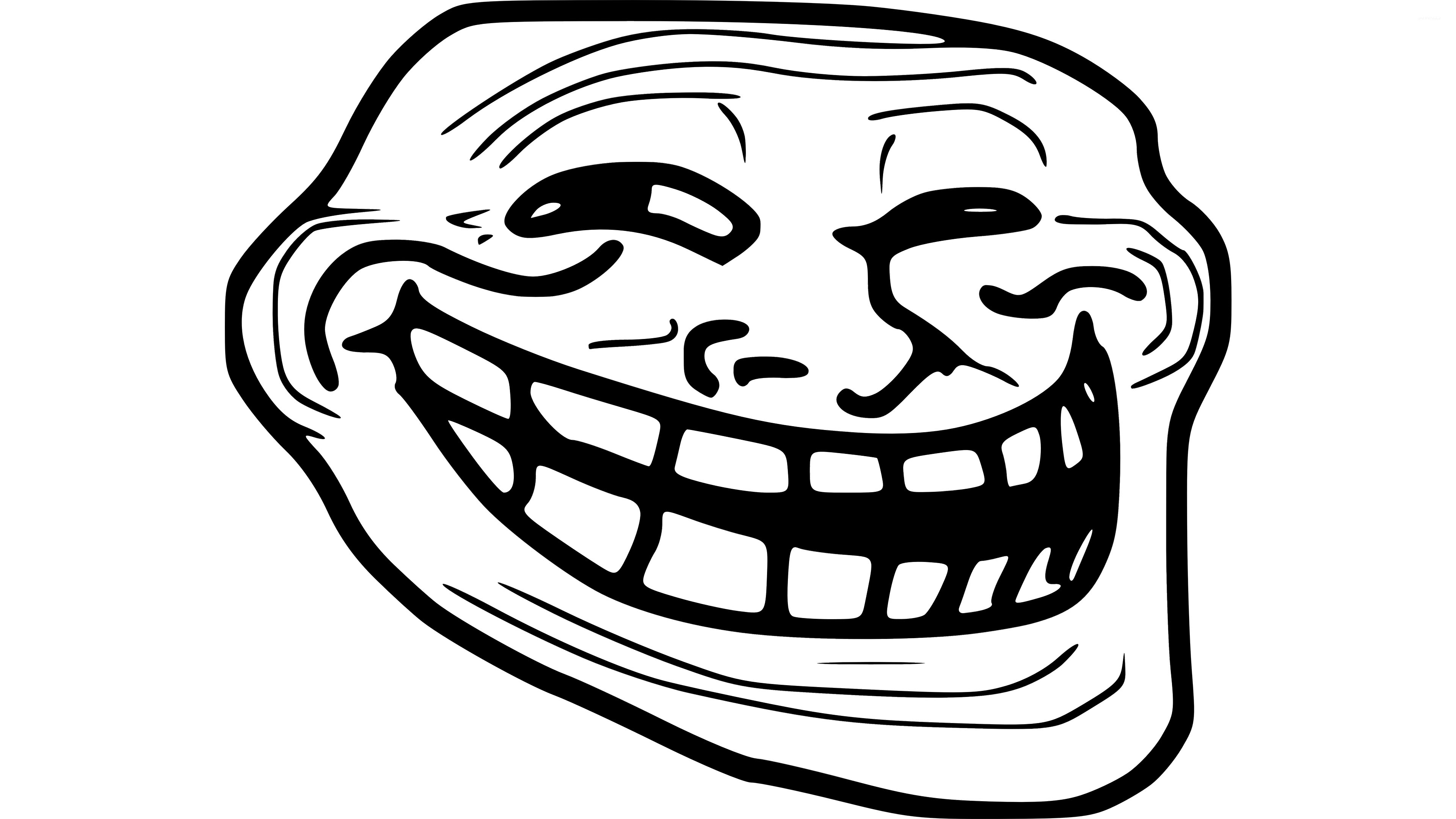
---
# Généralités sur Python : PIP
Installation :
```bash
sudo apt install python3-pip
```
Utilisation :
```bash
pip3 install truc # installe truc
pip3 uninstall machin # vire truc
pip3 freeze > requirements.txt # Sauvegarde les packages
# installés
pip3 install -r requirements.txt # Installe les packages
# listés dans requirements.txt
```
----
# Généralités sur Python : VirtualEnv
##### (ou comment ne pas polluer son PC)
Installation :
```bash
pip3 install virtualenv
```
Utilisation :
```bash
virtualenv env_formation
source env_formation/bin/activate
```
---
# Généralités sur Python : VirtualEnvWrapper
###### (réservé aux gens supérieurs sous linux)
Installation :
```bash
pip install --user virtualenvwrapper
```
Dans votre `.bashrc`
```bash
export WORKON_HOME=~/.virtualenvs
mkdir -p $WORKON_HOME
source ~/.local/bin/virtualenvwrapper.sh
```
Utilisation :
```bash
mkvirtualenv monprojet
workon monprojet
rmvirtualenv monprojet
```
---
# Mon premier site : Un blog
- Écrire des articles
- Lire des articles
----

---
# Comment démarrer un projet ?
Virtualenv :
```bash
cd là/où/vous/mettez/vos/projets/
virtualenv env_formation
source env_formation/bin/activate
```
VirtualenvWrapper :
```bash
mkvirtualenv env_formation
```
Création du projet :
```bash
pip install django
django-admin startproject mon_site
cd blog
./manage.py migrate
./manage.py runserver
```
---

---
# Comment démarrer un projet ?
Création de l'application :
```bash
./manage.py startapp blog
```
Enregistrement de l'application ( dans `mon_site/settings.py` ) :
```python
...
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'blog'
]
...
```
---
```bash
(env_formation) klafyvel@batman > ~/mon_site > tree
.
├── blog
│ ├── admin.py
│ ├── apps.py
│ ├── __init__ .py
│ ├── migrations
│ │ └── __init__ .py
│ ├── models.py
│ ├── tests.py
│ └── views.py
├── db.sqlite3
├── manage.py
└── mon_site
├── __init__ .py
├── __pycache__
│ ├── __init__ .cpython-36.pyc
│ ├── settings.cpython-36.pyc
│ └── urls.cpython-36.pyc
├── settings.py
├── urls.py
└── wsgi.py
```
---
# L'architecture MVT
Models Views Templates
---
## M comme Model
Les imports
```python
from django.db import models
```
---
## M comme Models
```python
class Article(models.Model):
"""Un article sur mon super site."""
text = models.TextField(verbose_name="Texte")
title = models.CharField(
max_length=255,
verbose_name="Titre"
)
date = models.DateField(
verbose_name="Date de parution"
)
def __str__ (self):
return "'{}' : {}".format(
self.title,
self.date
)
```
---
## Modifier la base de données
```bash
./manage.py makemigrations blog
./manage.py migrate
```
---
## Time to play !
```python
./manage.py shell
>>> from blog.models import Article
>>> a = Article()
>>> a
< Article: ' ' : None >
>>> from django.utils import timezone
>>> a.date = timezone.now()
>>> a.title = "Un super titre"
>>> a.text = "Un contenu vraiment très intéressant !"
>>> a
< Article: ' Un super titre ' : 2018-04-07 12:34:01 . 509609 + 00:00 >
>>> a.save()
```
---
## Time to play !
```python
>>> b = Article()
>>> b.title = "Un autre article"
>>> b.date = timezone.now()
>>> b.text = "Du contenu"
>>> b.save()
>>> Article.objects.all()
< QuerySet [ < Article: ' Un super titre ' : 2018-04-07 > ,
< Article: ' Un autre article ' : 2018-04-07 > ]>
```
```python
>>> Article.objects.get(pk=1)
< Article: ' Un super titre ' : 2018-04-07 >
>>> Article.objects.order_by('date')
< QuerySet [ < Article: ' Un super titre ' : 2018-04-07 > ,
< Article: ' Un autre article ' : 2018-04-07 > ]>
```
---
## Time to play !
```python
>>> import datetime
>>> d = datetime.timedelta(days=1)
>>> b.date += d
>>> b.save()
>>> Article.objects.filter(date__lte=timezone.now())
< QuerySet [ < Article: ' Un super titre ' : 2018-04-07 > ]>
```
---
# Mais quand est-ce qu'on affiche quelque chose dans le navigateur ?

---
# L'architecture MVT
## V comme Views
```python
from django.shortcuts import render
from django.http import HttpResponse
def index(request):
s = ("Bonjour et bienvenue"
"sur mon super site trop cool")
return HttpResponse(s)
```
---
## Routons mes bons
`blog/urls.py` (à créer) :
```python
from django.urls import path
from . import views
app_name = "blog"
urlpatterns = [
path('', views.index),
]
```
`mon_site/urls.py` :
```python
...
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('blog.urls')),
]
```
---
## Lancer le serveur :
```bash
./manage.py runserver
```
## Tadaaaa :

---
## Afficher des données !
```python
from django.shortcuts import render
from django.http import HttpResponse
from .models import Article
def index(request):
articles = Article.objects.order_by('-date')
s = ("Bonjour et bienvenue"
" sur mon super site trop cool"
"\nMes articles :"
)
for a in articles:
s += a.title + "\n"
return HttpResponse(s)
```
---
## Afficher des données !
### Votre site :

### Vous :

---
# L'architecture MVT
## T comme Templates
2018-04-10 09:28:27 +00:00
Dans `blog/templates/blog/list_articles.html` :
2018-04-07 13:49:12 +00:00
```html
< h3 > Liste des articles< / h3 >
{% for article in articles %}
< div >
< h4 > {{article.title}}< / h4 >
< p > Article écrit le {{article.date}}< / p >
{% endfor %}
```
2018-04-10 09:28:27 +00:00
---
## T comme Templates
Dans `blog/views.py` :
```python
from django.shortcuts import render
from django.http import HttpResponse
from .models import Article
def index(request):
articles = Article.objects.order_by('-date')
return render(
request,
'blog/list_articles.html',
{'articles':articles}
)
```
---
## Votre site :

---
## Vous :

---
## Étendre un template
Dans `templates/base.html` :
```html
<!DOCTYPE html>
< html >
< head >
< title > Mon super blog< / title >
< meta charset = "utf-8" / >
< / head >
< body >
< h1 > Mon super titre qu'on verra partout< / h1 >
{% block content %}{% endblock %}
< / body >
< / html >
```
---
## Étendre un template
Dans `mon_site/settings.py` (ligne 55):
```python
#...
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [os.path.join(BASE_DIR, 'templates')],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
#...
```
---
## Étendre un template
Dans `blog/templates/blog/list_articles.html`
```python
{% extends 'base.html' %}
{% block content %}
< h3 > Liste des articles< / h3 >
{% for article in articles %}
< div >
< h4 > {{article.title}}< / h4 >
< p > Article écrit le {{article.date}}< / p >
{% endfor %}
{% endblock %}
```
---
## Votre site

---
## Exercice : afficher un article
Objectif :

---
## Exercice : afficher un article
- Créer la vue dédiée (`def view_article(request, pk):`)
- La remplir (conseil regarder `django.shortcuts.get_object_or_404` )
2018-04-10 10:02:23 +00:00
- Créer l'url dédiée dans `blog/urls.py` (de la forme `article/<int:pk>` )
2018-04-10 09:28:27 +00:00
- Créer le template associé (dans `blog/templates/blog/view_article.html` )
---
## Ma solution
Dans `blog/views.py` :
```python
def view_article(request, pk):
article = get_object_or_404(Article, pk=pk)
return render(
request,
'blog/view_article.html',
{'article':article}
)
```
Dans `blog/urls.py` :
```python
path('article/< int:pk > ', views.view_article)
```
---
## Ma solution
Dans `blog/templates/blog/view_article.html` :
```html
{% extends 'base.html' %}
{% block content %}
< h2 > {{article.title}}< / h2 >
Publié le {{article.date}}.
< br / >
< br / >
{{article.text}}
{% endblock %}
```
---
## Tags
### → Commandes pour les templates
Exemple : `{% url %}`
Dans `blog/urls.py` :
```python
from django.urls import path
from . import views
app_name = "blog"
urlpatterns = [
path('', views.index, name="index"),
path(
'article/< int:pk > ',
views.view_article,
name="article"
)
]
```
---
## Tags
Dans `blog/templates/blog/list_articles.html` :
```html
{% extends 'base.html' %}
{% block content %}
< h3 > Liste des articles< / h3 >
{% for article in articles %}
< h4 >
< a href = "{% url 'blog:article' article.pk %}" >
{{article.title}}
< / a >
< / h4 >
< p > Article écrit le {{article.date}}< / p >
{% endfor %}
{% endblock %}
```
---
## Tags
Dans `templates/base.html` :
```html
<!DOCTYPE html>
< html >
< head >
< title > Mon super blog< / title >
< meta charset = "utf-8" / >
< / head >
< body >
< h1 > Mon super titre qu'on verra partout< / h1 >
< a href = "{% url 'blog:index' %}" >
Retour à l'accueil
< / a >
{% block content %}{% endblock %}
< / body >
< / html >
```
---

---

---
# Site admin
2018-04-10 10:02:23 +00:00
Enregistrer son modèle (dans `blog/admin.py` ) :
```python
from django.contrib import admin
from .models import Article
admin.site.register(Article)
```
Créer un superuser :
```bash
./manage.py createsuperuser
```
→ On peut éditer ses modèles ! rdv [http://127.0.0.1:8000/admin ](http://127.0.0.1:8000/admin )
2018-04-10 09:28:27 +00:00
---
2018-04-10 10:02:23 +00:00

---
# Quoi faire maintenant ?
- Formulaires
- Gestion des utilisateurs
- Les fichiers statiques (css)
- Le déploiement en production
- Les test unitaires :angel:
- ...
2018-04-10 09:28:27 +00:00
2018-04-10 10:02:23 +00:00
# >> docs.djangoproject.com <<
2018-04-10 11:23:12 +00:00
---

---

---

---

2018-04-07 13:49:12 +00:00
---
# Sites intéressants
- [Le blog Sam et Max ](http://sametmax.com )
- [Article sur virtualenv ](http://sametmax.com/les-environnement-virtuels-python-virtualenv-et-virtualenvwrapper/ )
- [Article sur Pip ](http://sametmax.com/votre-python-aime-les-pip/ )
- [Un autre article pour comprendre à quel point l'écosystème Python c'est le feu ](http://sametmax.com/creer-un-setup-py-et-mettre-sa-bibliotheque-python-en-ligne-sur-pypi/ )
- [La doc de Django ](http://docs.djangoproject.com/ )
- [Zeste de Savoir ](https://zestedesavoir.com/ )
- [Djangogirl ](https://tutorial.djangogirls.org/fr/ )
---
# Demander de l'aide :
- Vos Rézo(wo)mens :heart:
- IRC
- Telegram
- Mail
- Facebook
- Forums
- [Zeste de Savoir ](https://zestedesavoir.com/ )
- [Stack Overflow ](http://stackoverflow.com/ )